จากบทความที่ผ่านๆมา เป็นการปูพื้นก่อนที่จะนำ Graph Database มาใช้
ก่อนที่มาเริ่ม Coding ก็ให้มาติดตั้ง Environment ให้เสร็จเรียบร้อยก่อน ได้แก่
1. Java JDK
2. ติดตั้ง Path เป็น JAVA_HOME ให้เรียบร้อย
3. ติดตั้ง JAVA EE
4. ติดตั้ง Neo4j ให้เรียบร้อย
จากนั้นก็เริ่ม สร้าง Project ขึ้นมา select File -> new -> Java Project
จากนั้นก็เริ่ม Build Path ของ Neo4j เข้ามาใน Library
กด Properties
Add Library
User Library
New
ใส่ชื่อ
เลือก Library .jar
กด finish
เริ่มสร้าง “New -> Java Class”
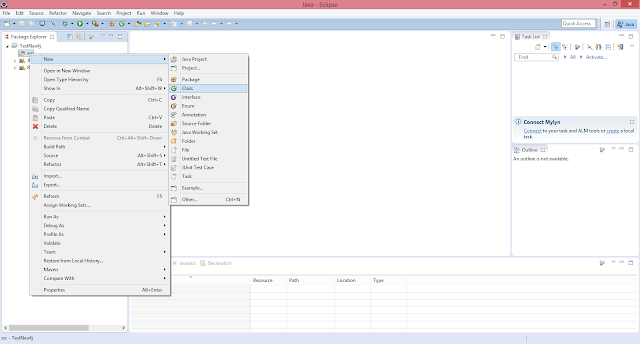
จากนั้นก็เริ่ม Import เข้ามาได้เลย
ในบทความนี้จะอธิบายตัวอย่างนี้เพียงคร่าวให้คุ้นชินกับคำสั่งก่อน
จากนี้ผมจะอธิบายความหมายของ คำสั่ง Code แต่ละบรรทัดให้อ่านกัน
หมายเหตุให้เติม /bin หลัง Neo4j_version ไปด้วย
ในส่วนนี้เป็นการสร้าง Path ของ Library โดยใส่ Path ที่ได้ Build Path เอาไว้
กำหนด Object ของ Graph database ได้แก่
Node Relation ดังที่ได้อธิบายไปบทความก่อนๆหน้านี้
และ GraphdatabaseService จะอธิบายในภายหลัง
Code ส่วนนี้เป็นการสร้าง Label ว่า RelTypes โดยใช้ Interface
ของNeo4j ชื่อ ReletionshipType ผ่านการใช้ enum โดยตั้งชื่อว่า KNOWS
Code ถัดไป คือการสร้างฐานข้อมูล โดยที่ใช้คำสั่ง graphDatabaseService = new GraphDatabaseFactory().newEmbeddedDatabase(Neo4j_DBPath);
ซึ่งจะต้องรวม Object - new GraphDatabaseFacetory().newEmbeddedDatabase("C:/Path Folder ที่เก็บฐานข้อมูล Graph");
ส่วน
first = graphDatabaseService.createNode();
first.setProperty("name", "SKLSongkiat");
second = graphDatabaseService.createNode();
second.setProperty("name", "Blog");
เป็นการสร้าง Node
และ
relation = first.createRelationshipTo(second, RelTypes.KNOWS);
relation.setProperty("relationship-type", "KNOWS");
เป็นการสร้าง Reletionship และใน Properties สร้างให้กับมันว่า KNOWS
จากนั้นก็ให้มันแสดงผล
Code ถัดไป เป็นการลบข้อมูล เช่น Node ต่างๆ
Method beginTX เป็น Method การเชื่อมต่อกับฐานข้อมูล
ปิด Database
และในส่วน Main ก็ นำMethod มาใช้
ผลลัพท์เมื่อรัน ก็จะได้แบบ Console ด้านล่าง
จริงๆแล้ว Code บางคำสั่งจะ deprecate ไปแล้ว ในบทหน้าจะเขียนอีกรูปหนึ่งให้ได้อ่านกัน
ลองนำ Source Code ด้านล่างไปลองทำตามดูก่อนครับ
Source CODE:
import org.neo4j.graphdb.Direction;
import org.neo4j.graphdb.GraphDatabaseService;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.Relationship;
import org.neo4j.graphdb.RelationshipType;
import org.neo4j.graphdb.Transaction;
import org.neo4j.graphdb.factory.GraphDatabaseFactory;
import org.neo4j.shell.impl.SystemOutput;
public class Helloworld {
//Path of Neo4j_Path
private static final String Neo4j_DBPath = "Users/Songkiat/Desktop";
// Create Parameter
Node first;
Node second;
Relationship relation;
GraphDatabaseService graphDatabaseService;
//list of relationship first KNOWS second
private static enum RelTypes implements RelationshipType{
KNOWS
}
void createDelete(){
//GraphDatabaseService
graphDatabaseService = new GraphDatabaseFactory().newEmbeddedDatabase(Neo4j_DBPath);
//Begin Transaction
Transaction transaction = graphDatabaseService.beginTx();
try{
//create Node & set Properties
first = graphDatabaseService.createNode();
first.setProperty("name", "SKLSongkiat");
second = graphDatabaseService.createNode();
second.setProperty("name", "Blog");
//Relationship
relation = first.createRelationshipTo(second, RelTypes.KNOWS);
relation.setProperty("relationship-type", "KNOWS");
//display
System.out.print(first.getProperty("name").toString() + " ");
System.out.print(relation.getProperty("relationship-type").toString());
System.out.println(" " + second.getProperty("name").toString());
//success transaction
transaction.success();
}
finally{
//finish the transaction
}
}
void removeData(){
Transaction transaction = graphDatabaseService.beginTx();
try{
//delete
first.getSingleRelationship(RelTypes.KNOWS,Direction.OUTGOING).delete();
System.out.println("nodes are remove");
//delete Node
first.delete();
second.delete();
transaction.success();
}finally{
//finish the transaction
transaction.finish();
}
}
void shutdown(){
//shutdown GraphDatabase
graphDatabaseService.shutdown();
System.out.println("Neo4j database is shutdown");
}
public static void main(String[] args) {
Helloworld hello = new Helloworld();
hello.createDelete();
hello.removeData();
hello.shutdown();
}
}
///////////////////////////////////////The End /////////////////////////////////////
REF:
http://technoracle.blogspot.com/2012/05/third-neo4j-tutorial-getting-started.html
http://neo4j.com/docs/stable/tutorials-java-embedded.html
http://neo4j.com/docs/stable/tutorials-java-embedded-hello-world.html
https://www.youtube.com/watch?v=Q8QCNcHnVS8
https://www.youtube.com/watch?v=1pKSWTzn0T4
https://www.youtube.com/watch?v=mq4Evw0EsQE
http://neo4j.com/api_docs//1.8.M01/